Deploy Next.js to Nginx server with GitHub
Author: Josh Mantei
Published: December 28, 2024
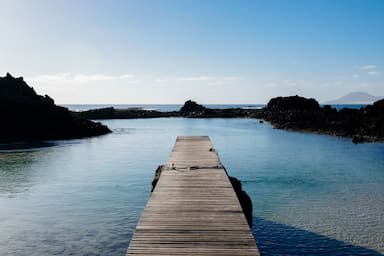
Use GitHub Actions to run a Next.js project on a Nginx web server.
Prerequisites
You will need a the username and IP address to your Nginx server. Additionally you will need your NextJS project deployed to GitHub to automate the deployment process.
Steps
1. Set up Communication between GitHub and Nginx via SSH
2. Set up PM2 Process Manager to run the NextJS Application
3. Configure Nginx to serve you new Nextjs application
4. Run NextJS app on the server
5. Create GitHub Actions Workflow to enable automatic deployment
1. Set up Communication between GitHub and Nginx via SSH
Generate a SSH key on your server:
cd ~/.ssh
ssh-keygen
This will generate two files:
- my-key (private key)
- my-key.pub (public key)
Next, create a config file :
nano config
Then, add the following lines for configuring your ssh connection to GitHub (the Host is what will replace github.com when cloning from GitHub and the Identity file is the private key with which you are connecting to GitHub):
# Default GitHub (git@)
Host my-host-name
HostName github.com
PreferredAuthentications publickey
IdentityFile ~/.ssh/my-key
Make sure that your private key is protected by running this command:
chmod 400 ~/.ssh/my-key
Next, add your public key to GitHub (This will allow your server to communicate with the GitHub repository):
- copy the public key from
my-key.pub
. - go to "Settings" and "SSH and GPG Keys" from the main navigation bar
- select "New SSH Key" and paste in the public key.
Then add the GitHub Actions secret to your NextJS repository:
- select "Settings", "Secrets and Variables" and then "Actions" for the desired NextJS repository. You will also need to do this for your
- HOST = your server's IP address.
- USERNAME = your server's username.
- SSH_PRIVATE_KEY = your server's SSH private key (the content of 'my-key').
These actions secrets will keep the data your use for connecting to the server private and secure.
Finally, we need to append our public key to the list of authorized keys inside the ~/.ssh
directory on the server to make sure the server recognises the key:
cat my-key.pub >> authorized_keys
2. Set up PM2 Process Manager to run the NextJS Application
install PM2:
sudo npm install -g pm2
Ensure PM2 starts on boot:
pm2 startup
Save the current PM2 processes:
pm2 save
This will make sure that your app is always running, even if there is an application or server reset.
3. Configure Nginx to serve you new Nextjs application
Depending on whether or not your website already exists you might already have files located in 'sites-available' and 'sites-enabled'.
Make sure that a configuration file for the Next.js application exists:
sudo nano /etc/nginx/sites-available/nextjs
Paste the following to the configuration file using the droplet IP address or your domain and the correct port (if default configuration already exists, just copy the part in the 'location' directive over to the file):
server {
listen 80;
server_name YOUR_IP_ADDRESS;
location / {
proxy_pass http://localhost:3000;
proxy_http_version 1.1;
proxy_set_header Upgrade $http_upgrade;
proxy_set_header Connection 'upgrade';
proxy_set_header Host $host;
proxy_cache_bypass $http_upgrade;
}
}
Make sure the file is linked to the site enabled directory:
sudo ln -s /etc/nginx/sites-available/nextjs /etc/nginx/sites-enabled/
Test configuration:
sudo nginx -t
Restart Nginx:
sudo service nginx restart
4. Run NextJS app on the server
Add 'ecosystem.config.js' file to the root of your NextJS project for the pm2 configuration:
module.exports = {
apps : [{
name : "nextjs-app",
script : "npm run start"
}]
Push new 'ecosystem.config.js' file to the GitHub repository.
Clone the repo to the desired location (normally to /var/www/my-website.com
):
git clone git@hostname:github-username/my-nextjs-repo.git
The 'hostname' is from the ssh config file that we set up earlier.
Now, we can start up the app using pm2:
cd my-nextjs-repo
npm install
npm run build
pm2 start --name 'nextjs-app' -- start
The name must match the name given to it in the 'ecosystem.config.js' file.
Save pm2 configuration changes:
pm2 save
Check pm2 status:
pm2 list
If configured correctly your app should now be live on the given domain or IP address.
5. Create GitHub Actions Workflow to enable automatic deployment
Add a 'your-branch-name.yml' file to the .github/workflows
directory in your repository with a customized repository name, branch name, application directory, and PM2 service name:
name: Deployment Workflow
# Trigger this workflow on pushes to the specified branch
on:
push:
branches:
- your-branch-name # The name of your branch goes here (e.g. main)
jobs:
deploy:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Install dependencies
run: npm install
- name: Build Next.js app
run: npm run build
- name: SSH Deploy
# Use the 'appleboy/ssh-action' action for SSH deployment
uses: appleboy/ssh-action@master
with:
host: ${{ secrets.HOST }} # Your server's IP address
username: ${{ secrets.USERNAME }} # Your server's username
key: ${{ secrets.SSH_PRIVATE_KEY }} # Your server's SSH private key
script: |
cd /path/to/your/application/directory # Specify the path to your app directory on the server
git pull
npm install
npm run build
pm2 restart nextjs-app # Replace nextjs-app with the name in ecosystem.config.js