Build a Frontend Mentor Project with React
Author: Josh Mantei
Published: February 9, 2025
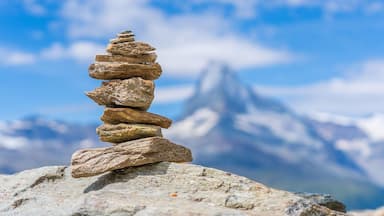
This is a walkthrough of how to built the Social links profile challenge from Frontend Mentor using React.
View the source code on GitHub.
Prerequisites
In order to build this project, you will need to have Node.js installed on your local system. You can create an account with Frontend Mentor if you want to following along with submitting your solution after you have built the project. The design files for this project can be downloaded from the Frontend Mentor Challenge page or from my GitHub repository. Additionally, if you are wanting to host your repository online you will need a GitHub account along with having Git installed on your local system.
Everything mentioned here is free to sign up for and to use for our purposes. If all you are wanting is to view and use the source code for your own projects your are free to do so at the GitHub repo link.
Steps
1. Create a React app using Vite
2. Download design assets from Frontend Mentor
3. Set up Git
4. Build Project
5. Add README
6. Deploy Project
1. Create a React app using Vite
We will be using Vite to create and built our React project.
To create the project, run the following command where you want the project to be stored:
npx create-vite@latest social-links-profile --template react
This will create a new directory called 'social-links-profile' and initialize it using the Vite template for React.
Next, install the project dependencies inside the project directory:
cd social-links-profile
npm install
Then, run the development server:
npm run dev
You should now be able to view the default Vite React template in your browser at http://localhost:5173/.
Congratulations! You have successfully set up a React app with Vite!
2. Download design assets from Frontend Mentor
Log in to Frontend Mentor and select "Start Challenge" on the Social Links Profile challenge page. Next, select "Download Starter" and your browser will automattically download a zip file.
Extract and open the downloaded "social-links-profile-main" directory.
Move the "preview.jpg" image and the "style-guide.md" file to the "design" directory and then move the "design" directory to the root of your react project.
Replace the "assets" directory that is located in the "src" directory in the root of your react project with the "assets" directory in the downloaded directory.
Keep the "index.html" file in the root of your react project to ensure that react is properly linked to the html. Just replace the following two tags inside the "Head":
<link rel="icon" type="image/svg+xml" href="/vite.svg" />
<title>Vite + React</title>
With the following from the downloaded "index.html":
<link
rel="icon"
type="image/png"
sizes="32x32"
href="./src/assets/images/favicon-32x32.png"
/>
<title>Frontend Mentor | Social links profile</title>
NOTE: "src/" was added to the file path in the href attribute for the link tag.
This will give your page the correct title and favicon when being displayed in the browser.
Next, copy the content of the ".gitignore" file from the downloaded files and paste it to the top of the ".gitignore" file inside the root of your project. Additionally, add another line to the file with the word "design". This will ensure that the design files will not be pushed to the remote repository.
Finally, create a "docs" directory in the root of your project and move the "README.md" and "README-template.md" files from the downloaded files to that directory. You can then delete the README.md inside the root of your project because it is now located in the "docs" directory.
Now, your development server will display an error at http://localhost:5173/ because of the removed "react.svg" image file from the "assets" directory. We will not be using the template code so we can therefore go ahead and remove the code related to that.
Begin by deleting the "App.css" and "index.css" files inside the "src" directory.
Then, remove lines 3 from "main.jsx":
import "./index.css";
Then, remove lines 1 to 4 and line 7 from "App.jsx":
import { useState } from "react";
import reactLogo from "./assets/react.svg";
import viteLogo from "/vite.svg";
import "./App.css";
const [count, setCount] = useState(0);
Then, replace lines 8 to 27 from "App.jsx":
<div>
<a href="https://vite.dev" target="_blank">
<img src={viteLogo} className="logo" alt="Vite logo" />
</a>
<a href="https://react.dev" target="_blank">
<img src={reactLogo} className="logo react" alt="React logo" />
</a>
</div>
<h1>Vite + React</h1>
<div className="card">
<button onClick={() => setCount((count) => count + 1)}>
count is {count}
</button>
<p>
Edit <code>src/App.jsx</code> and save to test HMR
</p>
</div>
<p className="read-the-docs">
Click on the Vite and React logos to learn more
</p>
With the boilerplate text from the downloaded "index.html" file:
Jessica Randall
London, United Kingdom
"Front-end developer and avid reader."
GitHub
Frontend Mentor
LinkedIn
Twitter
Instagram
Your development server at http://localhost:5173/ should now be working again and should just be displaying a white screen with the boilerplate text. The browser tab should be showing "Frontend Mentor | Social links profile" as well as the favicon. You might need to reload the browser window for these changes to take effect.
Last of all you can also remove the "vite.svg" image from the "public" directory as we will not be needing this anymore.
3 Set up Git
Now we can set up git to track the changes we make to the project as well as use it to upload our code to a remote GitHub repository when we are finished.
First we can initialize git inside the project directory:
git init
Check that git username and email match your GitHub account:
git config user.name
git config user.email
Then we can stage and commit the work we have done so far:
git add .
git commit -m"Initial commit;"
This is the only commit that will be included in the tutorial as commits can vary based on personal preference. Therefore, you can commit your own changes as you progress through the tutorial whenever you see fit. If you want, you can use the subtitles within the 'Build Project' section for your commits as a guide.
4 Build Project
Disable react prop-types
Add the following line to the 'rules' object in the 'eslint.config.js' file to disable prop-type checking:
export default [
{
rules: {
"react/prop-types": 0,
},
},
];
Add global CSS file
Add a 'reset.css' file to the 'src' directory to create a baseline style (Optional):
CSS resets by Josh Comeau and Andy Bell are two good options.
Add a 'global.css' file to the 'src' directory to add CSS that is for the entire application. This will include font and color initialization as well as layout and general styles for the project:
/* Fonts */
@font-face {
font-family: "Inter";
src: url(./assets/fonts/static/Inter-Regular.ttf);
font-weight: 400;
font-style: normal;
}
@font-face {
font-family: "Inter";
src: url(./assets/fonts/static/Inter-SemiBold.ttf);
font-weight: 600;
font-style: normal;
}
@font-face {
font-family: "Inter";
src: url(./assets/fonts/static/Inter-Bold.ttf);
font-weight: 700;
font-style: normal;
}
/* Colors */
:root {
/* PRIMARY */
--green: hsl(75, 94%, 57%);
--white: hsl(0, 0%, 100%);
/* SECONDARY */
--gray-700: hsl(0, 0%, 20%);
--gray-800: hsl(0, 0%, 12%);
--gray-900: hsl(0, 0%, 8%);
}
/* Layout */
#root {
min-height: 100vh;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
}
#root > * {
margin-top: auto;
margin-bottom: auto;
}
#root > footer:last-child {
margin-top: 0 !important;
margin-bottom: 0 !important;
}
/* General */
body {
font-family: "Inter", sans-serif;
background-color: var(--gray-900);
color: var(--white);
}
a {
color: inherit;
}
footer a:hover {
color: var(--green);
}
Don't forget to import these at the top of your 'App.jsx' file:
import "./global.css";
import "./reset.css";
Set up components
This project will be divided into three components: The first one is for the profile card as a whole. The second one is for the footer, and the third one is for the links inside the profile card.
To do this, we will create a 'components' directory inside the 'src' directory' and then add the directories for each component inside the 'components' directory:
src/components/ProfileCard
src/components/Footer
src/components/ProfileLink
Then each component directory will include a jsx file and a css module file that is linked to that jsx file:
For example, the 'ProfileCard' directory will contain a 'ProfileCard.jsx' and 'ProfileCard.module.css'.
Include the following boilerplate inside each component's jsx file and replace 'MyComponent' with the actual name of the component:
import styles from "./MyComponent.module.css";
function MyComponent() {
return <div></div>;
}
export default MyComponent;
Footer component
Add footer HTML and CSS for frontendmentor projects to 'Footer.jsx' and 'Footer.module.css'. Replace "MyUsername" with your name or Frontend Mentor username.
Footer.jsx:
import styles from "./Footer.module.css";
function Footer() {
return (
<footer className={styles.footer}>
<span>
Challenge by{" "}
<a
href="https://www.frontendmentor.io/?ref=challenge"
target="_blank"
className={styles.link}
>
Frontend Mentor
</a>
</span>
<span className={styles.divider}>│</span>
<span>
Coded by{" "}
<a
href="https://www.frontendmentor.io/profile/MyUsername"
target="_blank"
className={styles.link}
>
MyUsername
</a>
</span>
</footer>
);
}
export default Footer;
.footer {
margin-top: auto;
padding: 1rem;
font-size: 0.8rem;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
gap: 1rem;
}
.link {
color: inherit;
text-decoration: none;
}
.divider {
display: none;
}
@media (min-width: 640px) {
.footer {
flex-direction: row;
gap: 0.5rem;
}
.divider {
display: inline;
}
}
ProfileCard Component
This component uses props in order to simplify making changes to the data within the card. The childern of the component will be the ProfileLink components that we will create next.
import avatar from "../../assets/images/avatar-jessica.jpeg";
import styles from "./ProfileCard.module.css";
function ProfileCard({
children,
name = "Jessica Randall",
location = "London, United Kingdom",
description = "Front-end developer and avid reader.",
}) {
return (
<div className={styles.cardContainer}>
<div className={styles.card}>
<img className={styles.avatar} src={avatar} alt={`${name}'s avatar`} />
<div className={styles.infoContainer}>
<p className={styles.name}>{name}</p>
<p className={styles.location}>{location}</p>
</div>
<p className={styles.description}>"{description}"</p>
<div className={styles.linkContainer}>{children}</div>
</div>
</div>
);
}
export default ProfileCard;
.cardContainer {
padding: 1.5rem;
width: 100%;
max-width: 450px;
}
.card {
padding: 1.5rem;
background-color: var(--gray-800);
border-radius: 15px;
text-align: center;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
gap: 1.5rem;
}
.avatar {
border-radius: 999rem;
width: 90px;
}
.name {
font-size: calc((28 / 16) * 1rem);
font-weight: 600;
line-height: 1.1;
margin-bottom: 0.25rem;
}
.location {
color: var(--green);
font-size: calc((16 / 16) * 1rem);
font-weight: 600;
}
.description {
font-size: calc((14 / 16) * 1rem);
}
.linkContainer {
width: 100%;
display: flex;
flex-direction: column;
gap: 1rem;
}
@media (min-width: 640px) {
.card {
padding: 2.5rem;
}
.avatar {
width: 40%;
}
}
ProfileLink Component
This is a simple component containing a HTML link element with a hover effect.
import styles from "./ProfileLink.module.css";
function ProfileLink({ text = "GitHub", link = "https://github.com/" }) {
return (
<a className={styles.link} href={link} target="_blank">
{text}
</a>
);
}
export default ProfileLink;
.link {
text-decoration: none;
font-weight: 600;
font-size: calc((14 / 16) * 1rem);
background-color: var(--gray-700);
padding: 0.75rem;
border-radius: 7px;
transition: background-color 0.1s ease-in, color 0.1s ease-in;
display: block;
}
.link:hover {
background-color: var(--green);
color: var(--gray-900);
}
Add Components
Add the components to the 'App.jsx' file to include them in the project:
import "./global.css";
import "./reset.css";
import ProfileCard from "./components/ProfileCard/ProfileCard";
import ProfileLink from "./components/ProfileLink/ProfileLink";
import Footer from "./components/Footer/Footer";
function App() {
return (
<>
<ProfileCard
name="Jessica Randall"
location="London, United Kingdom"
description="Front-end developer and avid reader."
>
<ProfileLink text="GitHub" link="https://github.com/" />
<ProfileLink
text="Frontend Mentor"
link="https://www.frontendmentor.io/"
/>
<ProfileLink text="LinkedIn" link="https://www.linkedin.com/" />
<ProfileLink text="Twitter" link="https://x.com/" />
<ProfileLink text="Instagram" link="https://www.instagram.com/" />
</ProfileCard>
<Footer />
</>
);
}
export default App;
5 Add README
Inside your 'docs' directory, you can delete the 'README.md' file and then rename the 'README-template.md' file to 'README.md'.
Next you can take a screenshot of your project and add it to the 'docs' directory as 'screenshot.png'.
Your 'docs' directory should now have two files (README.md and screenshot.png).
Modify 'README.md' to fit your project development (Replace "MyUsername" with your GitHub and Frontend Mentor usernames.):
# Frontend Mentor - Social links profile solution
This is a solution to the [Social links profile challenge on Frontend Mentor](https://www.frontendmentor.io/challenges/social-links-profile-UG32l9m6dQ). Frontend Mentor challenges help you improve your coding skills by building realistic projects.
## Table of contents
- [Overview](#overview)
- [The challenge](#the-challenge)
- [Screenshot](#screenshot)
- [Links](#links)
- [My process](#my-process)
- [Built with](#built-with)
- [What I learned](#what-i-learned)
- [Author](#author)
## Overview
### The challenge
Users should be able to:
- See hover and focus states for all interactive elements on the page
### Screenshot

### Links
- Solution URL: [Add solution URL here]()
- Live Site URL: [Add live site URL here]()
## My process
### Built with
- [Vite](https://vite.dev/) - frontend build tool
- [React](https://react.dev/) - JS library
### What I learned
Using a component layout in which the component props can easily be changed at one location to make it easy to add custom data.
```jsx
<ProfileCard
name="Jessica Randall"
location="London, United Kingdom"
description="Front-end developer and avid reader."
>
<ProfileLink text="GitHub" link="https://github.com/" />
<ProfileLink text="Frontend Mentor" link="https://www.frontendmentor.io/" />
<ProfileLink text="LinkedIn" link="https://www.linkedin.com/" />
<ProfileLink text="Twitter" link="https://x.com/" />
<ProfileLink text="Instagram" link="https://www.instagram.com/" />
</ProfileCard>
```
## Author
- GitHub - [@MyUsername](https://github.com/MyUsername)
- Frontend Mentor - [@MyUsername](https://www.frontendmentor.io/profile/MyUsername)
6 Deploy Project
Create remote Repository
We will be using GitHub to host the local repository for the project.
Sign in to GitHub and then select the green "New" button under the "Repositories" tab.
Create a new repository with the following inputs:
- Repository name: Frontend-Mentor---Social-links-profile-solution---React
- Description: This is a solution to the Social links profile challenge on Frontend Mentor.
- Set visibility to "Public".
Push local repository to the remote repository
Replace MyUsername with your username.
git remote add origin git@github.com:MyUsername/Frontend-Mentor---Social-links-profile-solution---React.git
git branch -M main
git push -u origin main
If you refresh Github you should now see the code from your project in the remote repository.
Deploy live site
We will be using Netlify to deploy this project. To do this, you can link your GitHub account with Netlify by logging in to netlify with your Github account by selecting "Log in with Github" at https://www.netlify.com/login
Click "Add new site" on the "Sites" page in the Netlify control panel and then select "Import existing project".
Select "GitHub" to deploy your project with and then select the "Frontend-Mentor---Social-links-profile-solution---React" repository that you just created.
Set the Build command to "npm run build" and the Publish directory to "dist". Then select "Deploy" to deploy your project to a live site.
Your site will initialy be deployed to a randomly generated site name. To change this select the "Site configuration" option and then select "Change site name".
Enter "https://myusername-fm-social-links-profile.netlify.app" or your prefered custom url.
Submit solution to Frontend Mentor
Login to Frontend Mentor and search for "Social links profile" on the "Challenges"page.
You will now be taken to the challenge hub for that project. We have already completed steps 1 and 2. Select "Submit Solution" to begin step 3.
Enter "Social links profile" as the "Solution Title" and then copy the link of your remote GitHub repository to the "Repository URL" and the live site Netlify link to the "Live site URL".
Add the "Vite" and "React" tags to the project and then your have the option of filling out textfields answering questions about the development of your project before you select "Submit Solution".
Add Solution and Live site links to your README
Go to the "Links" section within your 'README.md' file and replace the placeholder text with the Solution and Live site links:
### Links
- Solution URL: [https://www.frontendmentor.io/solutions/social-links-profile-h_lE60EjwI](https://www.frontendmentor.io/solutions/social-links-profile-h_lE60EjwI)
- Live Site URL: [https://josh76543210-fm-social-links-profile.netlify.app/](https://josh76543210-fm-social-links-profile.netlify.app/)
Commit and push these changes to see them in the remote repository.
Congratulations! You have successfully built and deployed a project from Frontend Mentor using React.